Mastering React Series: useState Hook
useState
is a hook in React that allows you to add a state to your functional components. A state is an object that holds data that can change over time, such as user input or dynamic values, and it is used to render dynamic and interactive content in your component.Before the introduction of hooks in React, state management was limited to class components. With useState
, you can manage state in functional components, making it easier to manage and maintain your code.
Usage syntax :

Note: It’s a widely accepted and followed practice to destructure the returned array into named variables, such as something
and setSomething
, for better readability and ease of understanding. This is not a requirement, but a recommended best practice in the community.
Now let’s talk about its parameter and return value:
- Parameter :
initialState
: The initial value of the state. It can be a value of any type, but if you provide functions, it stores the value return value of that function, and also that function should be pure, and should take no arguments. - Return Value: It returns an array with exactly two values:
A.Current State
, for the initial render, this isinitialState
.
B.set
Function, that helps us to update the state and trigger the re-render of the component.
Let’s see a basic example of useState :
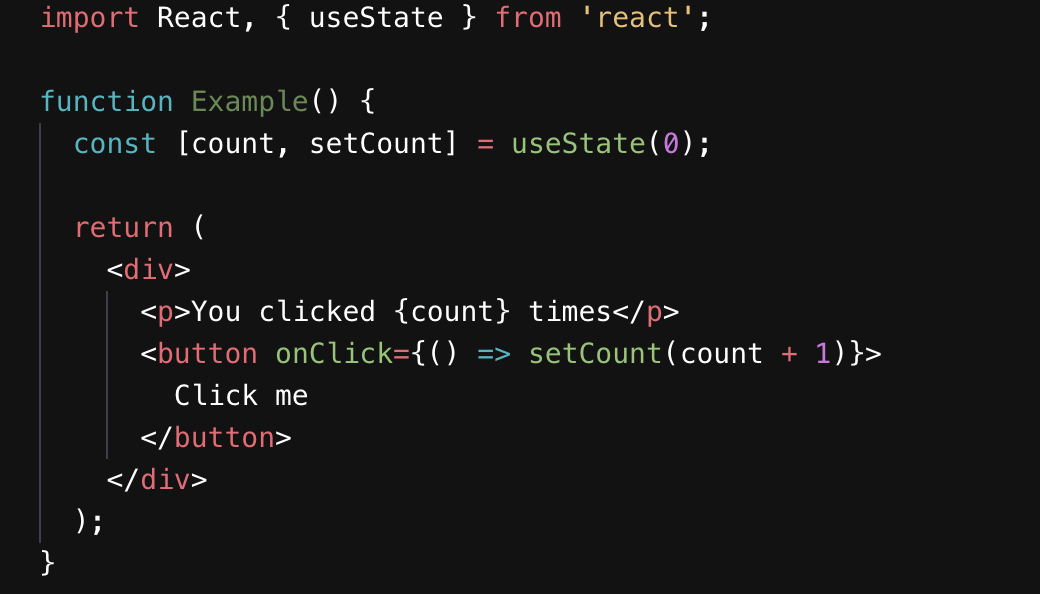
In the code above, useState
is imported from the React library. The useState
function returns an array with two elements: the current state value, and a function to update it. In this example, the state value is count
and the update function is setCount
.
The initial state value is passed as an argument to useState
(in this case, 0
), which represents the starting value for count
. When the button is clicked, setCount
is called with the updated value, incrementing the value of count
by 1. This causes React to re-render the component, displaying the updated value of count
.
You can have multiple states :
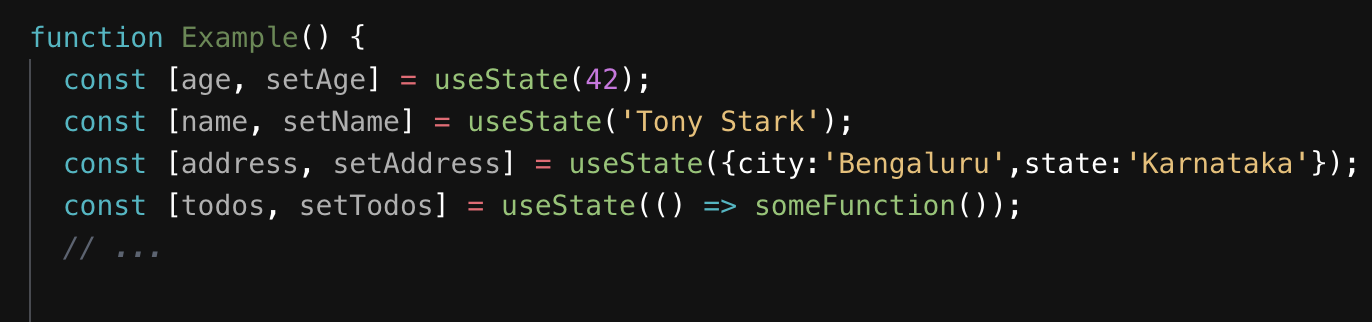
Set Function :
This function helps to update the state. It takes one parameter and returns no value. This parameter can be any value or it can be a function.
I. If it’s a value then it will simply update the state.
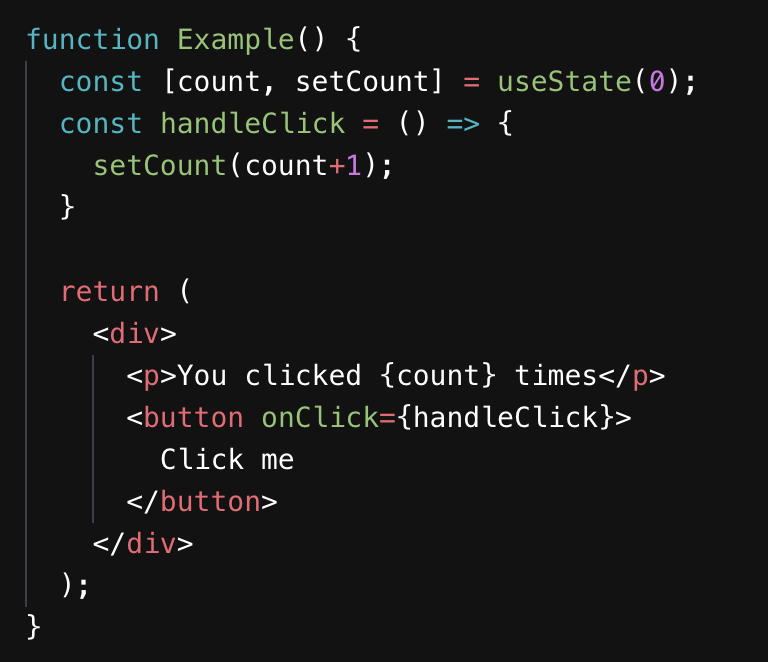
It’s important to note that React uses a concept called “reconciliation” to update the state, so it’s not recommended to modify the original state value directly. Instead, you should create a shallow copy of the value and update it.
For example, if you’re updating an array as the state value, you should use the spread
operator to create a new copy of the array with the updated values:

II. If you pass a function(Updater function) as an argument
The function is executed with the current state value as its argument, and its return value is used as the new state value.
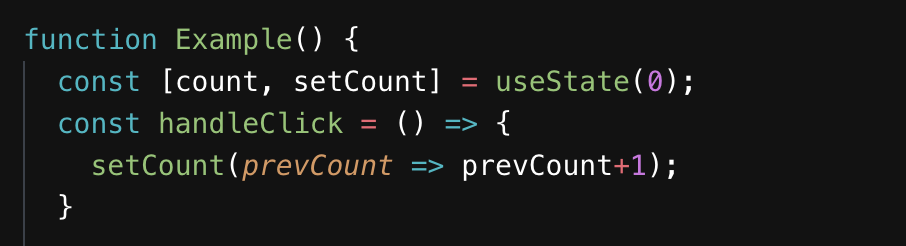
Why do we need these Updater function ?
In our example of incrementing count, if multiple clicks on the button happen before the first update to the state has finished, the state may end up in an unexpected state, as count + 1
will always be the same value regardless of how many clicks happened before the state update.There are many scenarios like that , few of them are :
This can be useful in a number of scenarios, such as:
- Preventing race conditions: If you need to update the state based on the current state value, using an updater function can help you avoid race conditions that may occur when updating the state directly. The updater function ensures that the state is updated atomically and consistently, even if the state changes between the render and the state update.
- Conditionally updating the state: You can use an updater function to conditionally update the state based on some condition. For example, you may want to update the state only if the current state value is less than a certain number.
- Updating complex state values: If your state value is an object or an array, you may want to update only certain properties or elements of the state, rather than replacing the entire state value. An updater function can help you make these updates in a controlled and optimized manner.
Important Point :
Calling the set
function does not change the current state in the already executing code. It only affects what useState
will return starting from the next render.

In conclusion, useState
is a powerful and essential hook in React that allows you to manage and update the state of your components. It helps you make your components dynamic and responsive to changes in the data, enabling you to build complex and interactive user interfaces.
Note: I believe in the power of hands-on learning, which is why I’ve chosen to provide images instead of code snippets in this tutorial. By writing down the code yourself, you’ll not only learn the syntax but also gain a deeper understanding of useState and strengthen your overall grasp of the subject.
Comments
Post a Comment